Java Help
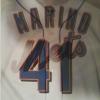
By
namarino
in Programming
in Programming
Go to solution
Solved by Evil Genius Jr.,
Yup! I just changed it to b<a instead of b<usernum.
-
Featured Topics
-
Topics
-
1
-
1
-
0
-
0
-
1
-
4
-
Merf01 ·
Posted in Power Supplies3 -
2
-
Shockboost99 ·
Posted in Power Supplies6 -
0
-
-
play_circle_filled
Latest From Linus Tech Tips:
I Will NOT Give You $250 for Your Broken Game - WAN Show April 26, 2024
-
play_circle_filled
Latest From ShortCircuit:
I tried 20 influencer foods, here are the best… and the worst…
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now