Help C++
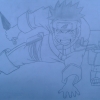
By
alphabeta
in Programming
in Programming
Go to solution
Solved by vm'N,
what pattern exactly ?
Psuedo-pattern:
*h*e*l*l*o*
-
Topics
-
Nexti88 ·
Posted in CPUs, Motherboards, and Memory1 -
HyperPro_Andrew118 ·
Posted in Graphics Cards1 -
tanjackson ·
Posted in New Builds and Planning3 -
7
-
rich_az ·
Posted in New Builds and Planning3 -
0
-
proxyyfps ·
Posted in Troubleshooting2 -
Krisp-kiwi ·
Posted in New Builds and Planning14 -
3
-
3
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now