C++ - Command line input parameter * (asterisk)
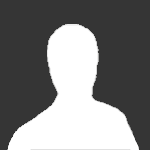
By
Guest Machines
in Programming
in Programming
Go to solution
Solved by rashdanml,
You'd need to find a way of escaping special characters using \. I'm not sure if C++ has a built in function to do that, so a way around it would be to loop through the provided input and replace known special characters with \[character].
-
Featured Topics
-
Topics
-
0
-
1
-
UnRaidLegends ·
Posted in Laptops and Pre-Built Systems0 -
2
-
7
-
2
-
Shane Martin ·
Posted in Displays2 -
5
-
INotPablo ·
Posted in CPUs, Motherboards, and Memory2 -
PatRed ·
Posted in New Builds and Planning8
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now