C++ Loops
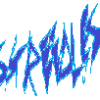
By
Skreedles
in Programming
in Programming
Go to solution
Solved by CornOnJacob,
It should be "firstnumber++" not "Firstnumber + firstnumber++". The ++ makes it go up by one, so you don't need more addition. You should also consider using a For loop:
for (i=firstnumber, i<=secondnumber, i++) { cout << i;}
Forgive my bad syntax. I haven't used C++ in a long while.
-
Featured Topics
-
Topics
-
Oufkiz ·
Posted in Programs, Apps and Websites0 -
16
-
ItsHunterM8 ·
Posted in Troubleshooting5 -
1
-
4
-
0
-
8
-
3
-
2
-
Worstcaster ·
Posted in Peripherals2
-
-
play_circle_filled
Latest From ShortCircuit:
I tried 20 influencer foods, here are the best… and the worst…
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now