Aligning an H1 and a <p> inside of a grid to be relative to each other no matter the screen size.
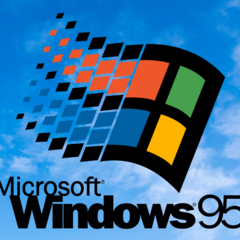
By
Tanaz
in Programming
in Programming
-
Featured Topics
-
Topics
-
0
-
JammJackGamer34 ·
Posted in New Builds and Planning0 -
cringowy ·
Posted in New Builds and Planning2 -
Pekokopiko ·
Posted in Graphics Cards0 -
1
-
deanx ·
Posted in Troubleshooting2 -
Bkstrat ·
Posted in Troubleshooting0 -
3
-
remo233 ·
Posted in Power Supplies6 -
2
-
-
play_circle_filled
Latest From ShortCircuit:
I tried 20 influencer foods, here are the best… and the worst…
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now