Game of Life GUI
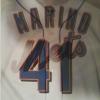
By
namarino
in Programming
in Programming
Go to solution
Solved by fizzlesticks,
This is what I've got. Unfortunately, the grid still does not resize with the window...really sorry to keep bothering you. Maybe my program design sucks or something.
You need to save the panel as an instance variable and get the width/height inside your drawGrid method then use those new values to calculate a new boxWidth.
-
Featured Topics
-
Topics
-
DreamCat04 ·
Posted in PC Gaming0 -
saltycaramel ·
Posted in Tech News1 -
3
-
1
-
1
-
1
-
VinSen ·
Posted in New Builds and Planning2 -
2
-
Fahizzle ·
Posted in CPUs, Motherboards, and Memory0 -
8
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now