I need some serious Java help.
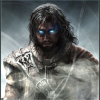
By
Voids
in Programming
in Programming
Go to solution
Solved by Samskip,
You should use if(s.equals("Square")).
== compares actual objects, .equals compares the actual string values.
-
Topics
-
0
-
avg boywithuke fan ·
Posted in Troubleshooting0 -
0
-
NetanelC ·
Posted in Troubleshooting0 -
0
-
5
-
3
-
4
-
3
-
0
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now