python Can someone modify this code with a start/stop interger and output the time it took to do the work?
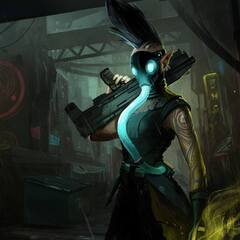
By
SVThuh
in Programming
in Programming
-
Featured Topics
-
Topics
-
joe_ollie909 ·
Posted in CPUs, Motherboards, and Memory4 -
DreamCat04 ·
Posted in PC Gaming0 -
saltycaramel ·
Posted in Tech News1 -
4
-
1
-
1
-
1
-
VinSen ·
Posted in New Builds and Planning4 -
2
-
Fahizzle ·
Posted in CPUs, Motherboards, and Memory0
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now