C++ modify vector of objects
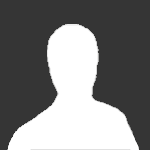
By
Guest
in Programming
in Programming
Go to solution
Solved by Sauron,
You need to reference the vector's object with a pointer, otherwise you're just modifying a local copy.
for (auto &i : vec) { ... }
-
Featured Topics
-
Topics
-
0
-
kennethk ·
Posted in Peripherals0 -
0
-
BTSHalfLifeAndGmodFan2003 ·
Posted in Storage Devices5 -
6
-
0
-
Abaris ·
Posted in Troubleshooting3 -
2
-
7
-
2
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now