How do i make an array thats given 2 values, add them and produce an array with results?
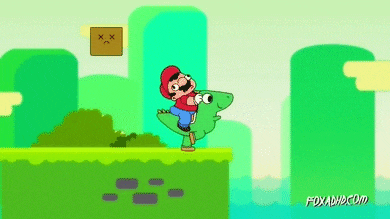
By
matin94
in Programming
in Programming
-
Featured Topics
-
Topics
-
0
-
1
-
alexfic ·
Posted in New Builds and Planning3 -
1
-
BryanOttawaDetroit ·
Posted in General Discussion1 -
2
-
2
-
GoStormPlays ·
Posted in WHALE LAN1 -
GoStormPlays ·
Posted in General Discussion6 -
9
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now