Inconsistent Unmanaged Memory C# behaviour
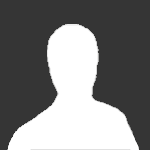
By
Guest
in Programming
in Programming
Go to solution
Solved by Guest,
I'm very drunk but something along the lines of
IntPtr ptrData = Marshal.AllocHGlobal(Marshal.SizeOf(clientToServerControllerInputPacket)); Marshal.StructureToPtr(clientToServerControllerInputPacket, ptrData, false);//
fixed it somewhere in the code with the pointer thingy...
-
Featured Topics
-
Topics
-
0
-
bluesheep ·
Posted in Troubleshooting0 -
0
-
0
-
0
-
RvG Iced ·
Posted in New Builds and Planning0 -
Rogers24 ·
Posted in Troubleshooting3 -
TurkishBTW ·
Posted in Peripherals0 -
1
-
kennethk ·
Posted in Peripherals1
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now