[ASMx86] How to move a user input array into another using Push and Pop.
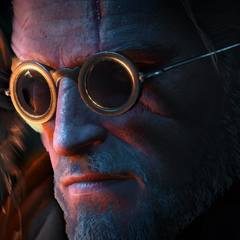
By
Noirgheos
in Programming
in Programming
-
Featured Topics
-
Topics
-
0
-
0
-
mbecker ·
Posted in Peripherals0 -
Prajnaanicca ·
Posted in CPUs, Motherboards, and Memory2 -
1
-
3
-
2
-
ZGruk ·
Posted in New Builds and Planning2 -
Cramig88 ·
Posted in Networking0 -
7
-
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Register a new accountSign in
Already have an account? Sign in here.
Sign In Now