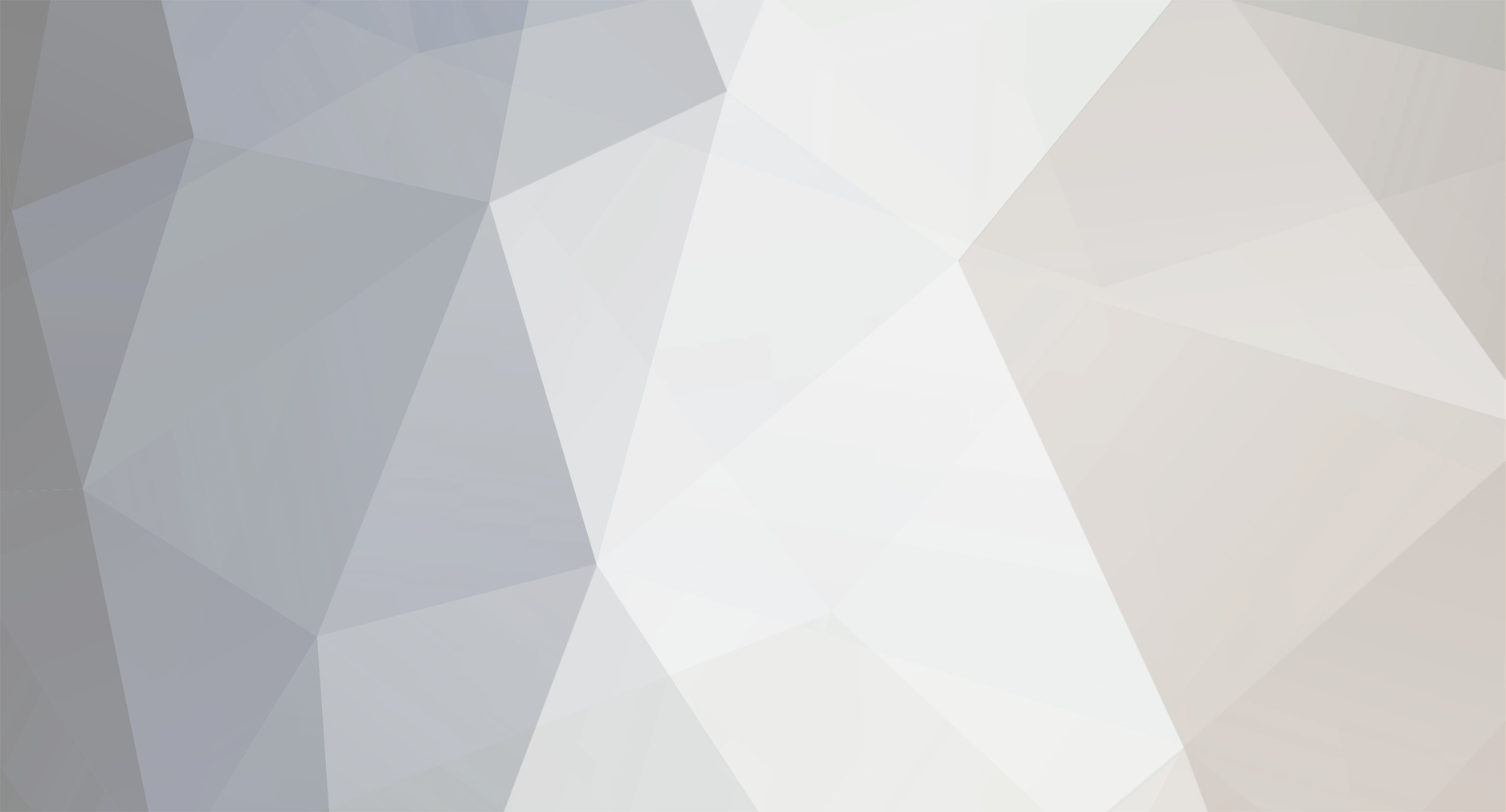
Dobri
Member-
Posts
6 -
Joined
-
Last visited
Awards
This user doesn't have any awards
Dobri's Achievements
-
This thread immediately made me think of a little gem I whipped up in a few hours trying to teach basic AI concepts to a few college kids. I build a travelling salesman genetic solver in JavaScript (and Python) and it works surprisingly well. When you add all the DOM crap it's something like 400 lines, full code (with demo) is on JSBin [edit link], feel free to fork it and do whatever, I'm all for it. Just ping me if you create something cool. I also took an image of a reasonably solved state (below) and the results aren't that unreasonable. Then, I bundled the basics into a Python class and put it on GitHub - the actual framework for the genetic solver is only 80 lines, including comments: import math import random class Organism: fitness = None data = None def __init__(self, data): self.data = data def mutate(self, mutate_func): self.data = mutate_func(self.data) def reproduce(self, reproduce_func, other): return Organism(reproduce_func(self.data, other.data)) def score(self, fitness_func): self.fitness = fitness_func(self.data) class GenerationStats: raw = [] def __init__(self, population): self.raw = map(lambda o: o.fitness, population.organisms) def percentile(self, p): return self.raw[int((len(self.raw)-1)/100*p)] class Population: generate_func = None mutate_func = None reproduce_func = None fitness_func = None organisms = [] state = None generation_data = [] def __init__(self, generate_func, mutate_func, reproduce_func, fitness_func, size): self.generate_func = generate_func self.mutate_func = mutate_func self.reproduce_func = reproduce_func self.fitness_func = fitness_func for i in range(0, size): self.organisms += [Organism(generate_func())] def step(self): # Score all organisms. for org in self.organisms: org.score(self.fitness_func) # Sort by fitness. self.organisms.sort(key=lambda o: o.fitness) # Record generation info. self.generation_data.append(GenerationStats(self)) # Kill with gradient. population_size = len(self.organisms) for i, org in enumerate(self.organisms): if random.randrange(population_size) < i: self.organisms[i] = None # Replace missing in population with new. org_copy = self.organisms[:] for i, org in enumerate(self.organisms): if org is None: mom = None dad = None while True: mom = self.organisms[random.randrange(population_size)] if mom is not None: break; while True: dad = self.organisms[random.randrange(population_size)] if dad is not None: break; org_copy[i] = dad.reproduce(self.reproduce_func, mom) org_copy[i].mutate(self.mutate_func) self.organisms = org_copy[:] def has_converged(self): top = self.generation_data[-1].percentile(0) median = self.generation_data[-1].percentile(50) # < 1% difference between top and median in population return abs((top-median)/top) < 0.01 You can use it by just telling it how to generate a random solution for your problem, what a success metric, how to mutate things and how to reproduce things and voila, it can solve any problem. Travelling salesman example is, again, in the repo but doesn't have that nice visual part JS version does. My favorite thing I did with this was to evolve the best convoluted neural network for a kinda specific image recognition task I had by training randomly structured networks and evaluating their accuracy as my "success" metric, then merging the successful ones based on a structural similarity function. Took a few days to run but I was super happy with the resulting network. I might still have the code somewhere if anyone cares to see it.
-
Help Writing a SimpleShell in Java (Not asking for code)
Dobri replied to BrownZeus's topic in Programming
A BufferedReader "Reads text from a character-input stream, buffering characters so as to provide for the efficient reading of characters, arrays, and lines." Use Java docs, they are your friends. Basically, you don't care about it and you think of it as a blackbox around the console input that you can call "readLine" on that will block until a line is provided, i.e. you press Enter. If you care about more details - System.in is the system's console input channel. InputStreamReader takes an input channel and creates a stream reader from it, i.e. you can call basic reads to fetch however many bytes are available at the time of the call. BufferedReader takes a StreamReader and buffers it for easier reading (so you can read lines and more efficiently read chunks, etc.) by simply adding a buffer and calling the StreamReader's basic read methods. WRT validation - I don't think your professor provides enough information for you to be able to know what command are or are not recognized (since the actual ProcessBuilder is given to you as a blackbox) but perhaps from the way you've described the problem he's asking you to validate there's exactly two things in the ArrayList you generate? So, for example, "bar foo" is a valid input but "bar foo biz" or "foo" are not. -
Except if you have good practices and split out your headers well so you can put all the usings you want in your cc because it will never get included anywhere. To answer the original topic without starting a flame war, I have seldom seens newbies do well with C++/Java/C#/<insert favorite heavily OOP language here> as their first language. You can learn C but why. I did the mistake of trying to force C++ down beginner programmer's throats because "it's the way you gain deeper knowledge". It's also the way you get so overwhelmed by everything and give up. A good starting point is a scripting language. Since you already have Python knowledge, I'd stick with that and it's a pretty solid language. Another thing you can try is Go (to a point). It's kind of a nice middle ground between scripting and production coding. If you really hate yourself or are interested in web, you can also give Ruby or PHP a shot + JavaScript to either one. You'd be able to build pretty robust web solutions in only a few weeks of coding and you'd feel very empowered. You can even try Node.js so you can learn just one language - JavaScript - and do both server and client code. Aaaand with all of the above said, just pick something you like and enjoy. If you're a Lisp person or a Haskell or even a Brainf* person, no one will judge you, it's really cool you want to get to coding. Aiming for something that will "look good on a resume" or "is the right way to do things" won't really get you anywhere. Specifically by the things you outlined, I'd recommend you get some PERL or Python and learn bash (not really a language but a useful skill nonetheless).
-
Help Writing a SimpleShell in Java (Not asking for code)
Dobri replied to BrownZeus's topic in Programming
Not exactly, List is an interface and ArrayList is a class than implements the interface. So the method will work as is. Easiest way you can think of it is List is a contract that says any "List" has to have these methods. In order for ArrayList to call itself a list, it has to abide by that contract but that's irrelevant to you - you're saying I'll take any List and your instructor is saying here's an ArrayList. Since it has all the methods, it will just work with your code as long as you stick to using List methods. Yeah, basically what he's asking is to split up the command, put all the stuff in an ArrayList and pass it on to the ProcessBuilder. That's pretty much it. Since you're already reading the line, it's a matter of figuring out how to split a string and put in an ArrayList - since you mentioned you're not looking for code, I'll let you take the reins on this one from here -
Weird Temperature Spikes after OC 6800k
Dobri replied to Dobri's topic in CPUs, Motherboards, and Memory
Hm, yeah, that's a good point. I guess I was mistaking processor use with the nature of the operations, I can see some prime95 cycles being more straneous on the CPU, even though the net "used cycles" does not change. That doesn't explain why I haven't seen it before but I'll just assume I didn't have a benchmark running long enough, considering I haven't tried OC the 6800 before. That still leaves the open question - CPU was stable at stock clock for months. Why is it bringing it down to stock clock now makes the PC crash while gaming? And in general, what can I do to make the CPU stable, at any clock speed but preferrably ~4.1 which seems to peak in the high 80 during one of those spikes but runs at low 70 at full load otherwise? I know 100C is high but I don't think it's high enough to cause permanent damage to the CPU, especially for the 2 minutes it thermal throttled for and considering specs say 105 to be the T max, which is supposedly the maximum temperature before lifetime is significantly affected. That's exactly what I did that I perceive caused all of this. Auto overclock bricked Windows (couldn't even boot safe mode or run any repairs, literally had to reinstall). I promised myself I would not use it again until everyone starts badgering me how much better it is or manual OC is turned down completely, whichever comes first. And I meant I wasn't looking for the usual "You don't need two 1080s and you definitely don't need a 6800 for gaming" opinion some people have a hard time keeping to themselves. Compliments on its ballerness are completely welcome, I'd do full specs but you already know the good stuff. -
OK, so this is a weird and kinda lengthy one so please stay with me. Situation: Bought baller PC a few months ago, 2x1080, 6800k, all the good stuff (not interested in opinions). Overclocked 1080 to 2050 stable - success. Tried Asus software overclock for the 6800k, bricked my windows, cursed at them, figured it's crap and decided I'll do it manually later. Fast forward several months of heavy use with no issues. I decide one day "Oh hey, let's go back to that overclock". Overclock it to ~4.3GHz with a AIO water cooler. Success. Temps ~80 at full load on all 6 cores, messed around with individual core clocks to tighten the temp range, all is well. Stress test ~30 minutes, perfectly stable. Alright, let's get to gaming. Game for a few hours, computer freezes. Force reboot, Asus says "Overclocking failure". Well that's weird, let's dial it back a bit. Doesn't matter, won't turn on, "overclocking failure" gone, post code 61 (NVRAM Failure). Freaking out, shut down computer. Turn it back on in 5 minutes, boots up just fine to Windows. OK, that was weird, let's stress test again. This time, I see these huge (15-20C) temperature spikes in the middle of stress testing (100% load throughout, no clock change but temps spike). Poking around at Hardware Monitor, I see matching spikes in power. Voltage - stable. Power - spikes from 90-ish reading to 120-ish reading for no reason (probably not real watts but that's what HW monitor was saying). Oh, maybe I have a crappy PSU. I was going to change it anyway so I bought a new one, well capable of supporting the 2x1080 and the 6800k + whatever other residual load. Same weird power + temp spikes. Play around with digi+ vrm settings to turn off all auto-power management and basically set everything to OC settings (disabled eco stuff, enabled power stuff). Hardware Monitor no longer reads Power but temps continue to spike. All throughout this experimentation rig is stable during 100% load in stress test but crashes in gaming load (???) with that same "overclocking failure" + post 61 + wait 5 minutes to use your PC. I've dialed it all the way back to stock clock and it still crashes while gaming, despite stable results during stress test (outside temp spikes). Oh and water temp never gets above the mid-40s (reported by Corsair Link) even after ~30 minutes of stress test. Any ideas? I've attached the best graph I have of this in action, clock vs tmp of both the 6800k and 2x1080 while running prime95 and furmark. I saw them being stable at the start so I went ahead and did something else for a while or I would've stopped the test at the 100C (!!!!) peak for the CPU.